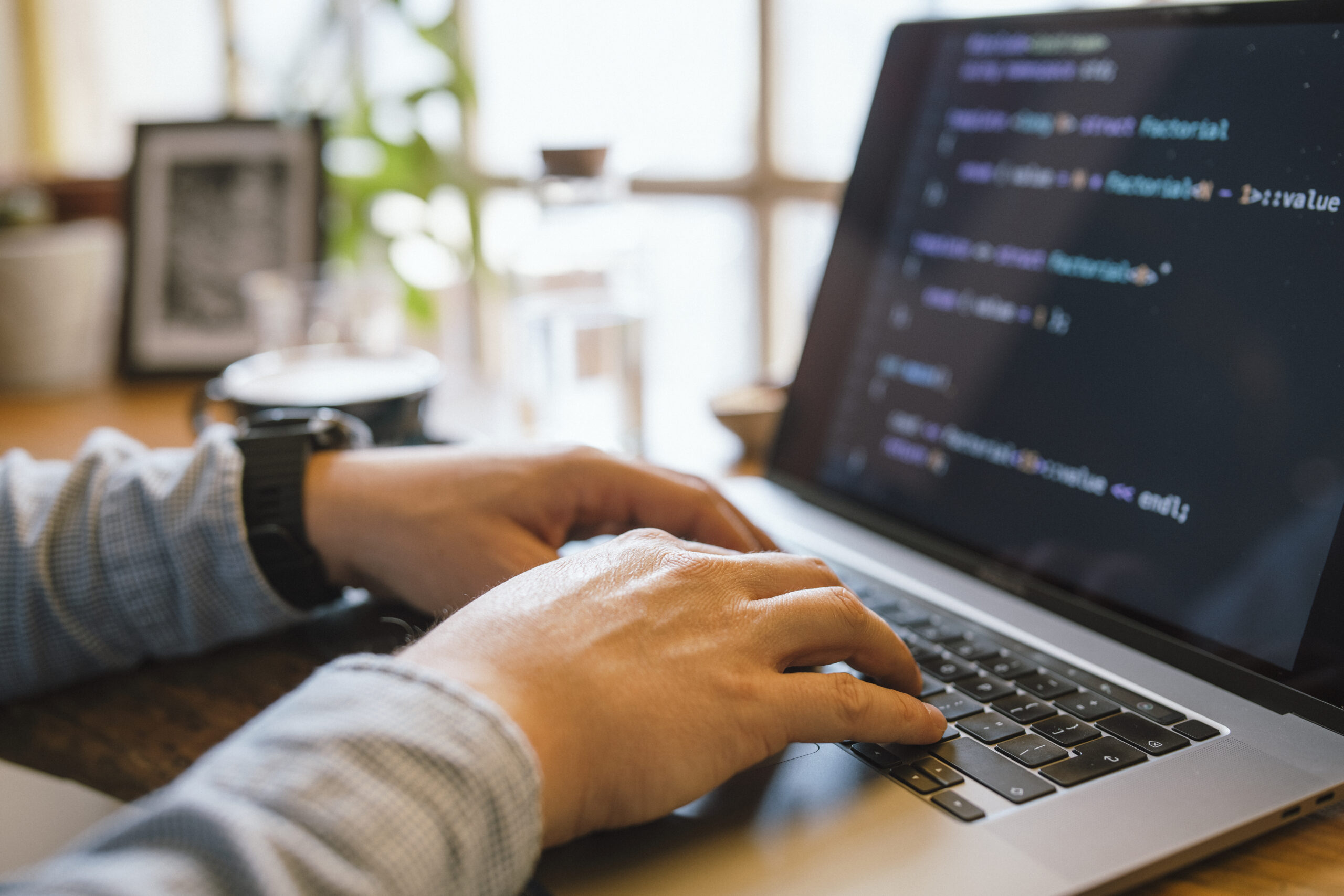
Debugging is one of the most vital — yet frequently disregarded — techniques inside of a developer’s toolkit. It's not nearly fixing broken code; it’s about knowing how and why factors go Erroneous, and Discovering to think methodically to solve issues effectively. No matter if you are a rookie or maybe a seasoned developer, sharpening your debugging abilities can conserve hrs of disappointment and substantially increase your productiveness. Allow me to share many techniques to aid developers level up their debugging game by me, Gustavo Woltmann.
Learn Your Instruments
One of several quickest techniques developers can elevate their debugging skills is by mastering the tools they use every day. Though crafting code is one particular Portion of advancement, understanding how you can connect with it properly in the course of execution is equally significant. Present day advancement environments come Outfitted with potent debugging abilities — but a lot of developers only scratch the area of what these equipment can do.
Acquire, such as, an Built-in Improvement Ecosystem (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These tools help you set breakpoints, inspect the worth of variables at runtime, stage via code line by line, as well as modify code around the fly. When used the right way, they Allow you to notice just how your code behaves in the course of execution, which is priceless for tracking down elusive bugs.
Browser developer instruments, including Chrome DevTools, are indispensable for entrance-stop builders. They help you inspect the DOM, check community requests, check out genuine-time performance metrics, and debug JavaScript from the browser. Mastering the console, sources, and community tabs can change disheartening UI troubles into workable jobs.
For backend or system-stage builders, tools like GDB (GNU Debugger), Valgrind, or LLDB offer you deep Command above running processes and memory administration. Discovering these resources can have a steeper Studying curve but pays off when debugging effectiveness problems, memory leaks, or segmentation faults.
Over and above your IDE or debugger, come to be comfy with Edition Regulate systems like Git to grasp code record, find the exact minute bugs were being introduced, and isolate problematic variations.
In the end, mastering your equipment usually means going further than default configurations and shortcuts — it’s about creating an intimate knowledge of your advancement natural environment to make sure that when concerns come up, you’re not missing in the dead of night. The greater you are aware of your applications, the greater time you could spend resolving the particular challenge rather then fumbling through the procedure.
Reproduce the condition
One of the most crucial — and often ignored — actions in successful debugging is reproducing the challenge. Just before jumping to the code or producing guesses, developers need to produce a dependable ecosystem or circumstance wherever the bug reliably appears. With out reproducibility, fixing a bug becomes a activity of probability, typically leading to squandered time and fragile code alterations.
The first step in reproducing a dilemma is collecting as much context as is possible. Check with inquiries like: What actions led to The difficulty? Which surroundings was it in — advancement, staging, or manufacturing? Are there any logs, screenshots, or mistake messages? The greater detail you have got, the less complicated it gets to be to isolate the precise problems below which the bug takes place.
As soon as you’ve collected more than enough info, seek to recreate the trouble in your local setting. This could signify inputting the identical details, simulating equivalent person interactions, or mimicking program states. If The difficulty appears intermittently, take into consideration creating automated assessments that replicate the edge scenarios or state transitions included. These tests not merely help expose the challenge but also avoid regressions Sooner or later.
Occasionally, The problem may very well be atmosphere-distinct — it'd happen only on specific running units, browsers, or below certain configurations. Working with tools like virtual devices, containerization (e.g., Docker), or cross-browser tests platforms may be instrumental in replicating this sort of bugs.
Reproducing the situation isn’t simply a step — it’s a attitude. It calls for tolerance, observation, in addition to a methodical approach. But when you can constantly recreate the bug, you happen to be by now midway to correcting it. That has a reproducible state of affairs, you can use your debugging tools much more efficiently, examination prospective fixes securely, and talk far more Obviously using your staff or buyers. It turns an summary criticism right into a concrete obstacle — Which’s the place developers thrive.
Read through and Fully grasp the Error Messages
Error messages tend to be the most respected clues a developer has when some thing goes wrong. Rather than looking at them as discouraging interruptions, builders must discover to treat mistake messages as immediate communications from your method. They normally show you just what exactly took place, in which it happened, and in some cases even why it took place — if you understand how to interpret them.
Start by studying the information meticulously and in comprehensive. A lot of developers, specially when underneath time stress, glance at the main line and promptly commence making assumptions. But further inside the mistake stack or logs could lie the true root bring about. Don’t just copy and paste mistake messages into serps — study and have an understanding of them very first.
Break the error down into parts. Could it be a syntax mistake, a runtime exception, or possibly a logic mistake? Does it place to a particular file and line selection? What module or operate triggered it? These issues can manual your investigation and place you toward the dependable code.
It’s also helpful to grasp the terminology from the programming language or framework you’re employing. Error messages in languages like Python, JavaScript, or Java usually abide by predictable patterns, and Mastering to recognize these can dramatically increase your debugging procedure.
Some problems are imprecise or generic, and in Individuals instances, it’s important to examine the context during which the mistake happened. Check relevant log entries, enter values, and up to date modifications while in the codebase.
Don’t forget about compiler or linter warnings either. These typically precede greater challenges and provide hints about probable bugs.
In the end, mistake messages are usually not your enemies—they’re your guides. Studying to interpret them accurately turns chaos into clarity, encouraging you pinpoint troubles a lot quicker, minimize debugging time, and turn into a more productive and self-assured developer.
Use Logging Properly
Logging is one of the most strong tools inside of a developer’s debugging toolkit. When utilized properly, it offers true-time insights into how an software behaves, encouraging you comprehend what’s happening under the hood without needing to pause execution or step throughout the code line by line.
A superb logging tactic starts with understanding what to log and at what level. Typical logging ranges consist of DEBUG, Information, WARN, Mistake, and Lethal. Use DEBUG for in-depth diagnostic facts all through progress, Details for standard activities (like productive commence-ups), WARN for potential issues that don’t crack the applying, ERROR for real problems, and FATAL in the event the process can’t proceed.
Stay away from flooding your logs with excessive or irrelevant details. An excessive amount logging can obscure crucial messages and slow down your method. Focus on vital gatherings, condition modifications, enter/output values, and critical conclusion factors inside your code.
Structure your log messages Obviously and consistently. Include things like context, including timestamps, ask for IDs, and function names, so it’s easier to trace troubles in distributed devices or multi-threaded environments. Structured logging (e.g., JSON logs) could make it even much easier to parse and filter logs programmatically.
In the course of debugging, logs Permit you to observe how variables evolve, what conditions are fulfilled, and what branches of logic are executed—all with out halting This system. They’re Particularly precious in creation environments exactly where stepping as a result of code isn’t doable.
In addition, use logging frameworks and applications (like Log4j, Winston, or Python’s logging module) that assistance log rotation, filtering, and integration with checking dashboards.
In the end, clever logging is about balance and clarity. By using a well-imagined-out logging solution, you'll be able to lessen the time it will take to spot difficulties, gain deeper visibility into your purposes, and Increase the All round maintainability and dependability of your code.
Consider Similar to a Detective
Debugging is not just a specialized process—it is a form of investigation. To efficiently discover and take care of bugs, builders should strategy the method similar to a detective resolving a mystery. This attitude will help stop working elaborate issues into manageable sections and follow clues logically to uncover the root result in.
Start off by accumulating proof. Think about the indications of the problem: error messages, incorrect output, or efficiency troubles. The same as a detective surveys a criminal offense scene, accumulate just as much appropriate facts as you may without the need of leaping to conclusions. Use logs, take a look at scenarios, and consumer studies to piece collectively a clear image of what’s happening.
Next, kind hypotheses. Request oneself: What could possibly be leading to this behavior? Have any changes recently been built into the codebase? Has this challenge transpired just before below equivalent circumstances? The goal should be to slim down prospects and determine opportunity culprits.
Then, take a look at your theories systematically. Try and recreate the trouble in a managed surroundings. In the event you suspect a selected operate or component, isolate it and validate if The problem persists. Similar to a detective conducting interviews, question your code queries and Enable the final results lead you nearer to the truth.
Pay back near attention to smaller information. Bugs often cover inside the the very least anticipated locations—similar to a missing semicolon, an off-by-a person error, or a race affliction. Be comprehensive and affected individual, resisting the urge to patch The problem without entirely comprehending it. Momentary fixes could cover the real dilemma, only for it to resurface later on.
Last of all, preserve notes on what you experimented with and acquired. Just as detectives log their investigations, documenting your debugging course of action can save time for foreseeable future issues and aid Many others realize your reasoning.
By imagining similar to a detective, developers can sharpen their analytical capabilities, solution issues methodically, and turn into more practical at uncovering hidden concerns in elaborate methods.
Publish Checks
Writing exams is among the simplest methods to boost your debugging techniques and In general development effectiveness. Assessments don't just help catch bugs early but in addition serve as a safety Internet that provides you self esteem when earning modifications towards your codebase. A well-tested application is easier to debug because it enables you to pinpoint precisely in which and when a difficulty happens.
Begin with unit tests, which concentrate on person functions or modules. These small, isolated tests can quickly expose whether a selected bit of logic is Performing as predicted. Each time a examination fails, you right away know in which to appear, considerably reducing some time expended debugging. Unit exams are Particularly useful for catching regression bugs—challenges that reappear immediately after Beforehand currently being set.
Subsequent, combine integration assessments and stop-to-finish checks into your workflow. These enable be certain that numerous parts of your software perform together effortlessly. They’re notably helpful for catching bugs that manifest in intricate methods with various parts or solutions interacting. If a little something breaks, your assessments can tell you which Component of the pipeline failed and underneath what situations.
Crafting exams also forces you to definitely Consider critically about your code. To test a feature adequately, you'll need to be familiar with its inputs, anticipated outputs, and edge conditions. This amount of being familiar with In a natural way potential customers to higher code composition and fewer bugs.
When debugging a concern, writing a failing examination that reproduces the bug is usually a powerful starting point. Once the examination fails continuously, you'll be able to deal with fixing the bug and look at your exam pass when The problem is solved. This approach ensures that precisely the same bug doesn’t return Down the road.
In brief, composing checks turns debugging from the irritating guessing match right into a structured and predictable system—assisting you catch additional bugs, faster and much more reliably.
Just take Breaks
When debugging a tough difficulty, it’s simple to become immersed in the challenge—observing your monitor for several hours, trying Answer right after Option. But Just about the most underrated debugging equipment is actually stepping absent. Getting breaks can help you reset your head, cut down irritation, and infrequently see The difficulty from the new standpoint.
If you're much too near the code for too long, cognitive tiredness sets in. You could commence overlooking clear problems or misreading code which you wrote just hrs earlier. Within this state, your Mind results in being fewer efficient at problem-resolving. A brief stroll, a coffee crack, or maybe switching to a unique process for 10–15 minutes can refresh your focus. Lots of builders report obtaining the root of a dilemma when they've taken time for you to disconnect, letting their subconscious work during the qualifications.
Breaks also aid stop burnout, Primarily through more time debugging sessions. Sitting down in front of a screen, mentally caught, is not just unproductive but will also draining. Stepping absent permits you to return with renewed energy and also a clearer frame of mind. You may instantly detect a missing semicolon, a logic flaw, or simply a misplaced variable that eluded you ahead of.
In the event you’re trapped, an excellent general guideline is usually to set a timer—debug actively for forty five–60 minutes, then have a 5–ten moment split. Use that point to move about, extend, or do one thing unrelated to code. It may well come to feel counterintuitive, Particularly underneath tight deadlines, but it surely really causes quicker and more practical debugging In the end.
Briefly, taking breaks just isn't an indication of weakness—it’s a wise system. It provides your Mind House to breathe, enhances your perspective, and aids you steer clear of the tunnel vision That usually blocks your development. Debugging is actually a psychological puzzle, and relaxation is part of fixing it.
Learn From Every single Bug
Each individual bug you come across is much more than simply a temporary setback—It really is a chance to mature as being a developer. No matter if it’s a syntax mistake, a logic flaw, or perhaps a deep architectural situation, every one can instruct you one thing precious if you make an effort to read more mirror and examine what went Erroneous.
Get started by asking by yourself some vital questions once the bug is resolved: What triggered it? Why did it go unnoticed? Could it are already caught previously with greater techniques like device screening, code opinions, or logging? The responses generally expose blind spots in your workflow or comprehending and assist you to Construct more powerful coding routines moving forward.
Documenting bugs will also be a wonderful pattern. Continue to keep a developer journal or maintain a log in which you Observe down bugs you’ve encountered, the way you solved them, and Whatever you realized. With time, you’ll start to see styles—recurring difficulties or prevalent problems—which you can proactively stay away from.
In team environments, sharing what you've acquired from the bug along with your peers is usually In particular effective. Whether or not it’s via a Slack concept, a short write-up, or A fast information-sharing session, helping Many others stay away from the exact same difficulty boosts crew efficiency and cultivates a much better Finding out culture.
Additional importantly, viewing bugs as lessons shifts your mentality from stress to curiosity. Rather than dreading bugs, you’ll get started appreciating them as vital parts of your progress journey. In any case, a few of the most effective developers are certainly not the ones who produce ideal code, but individuals that constantly master from their blunders.
Eventually, Every bug you deal with adds a whole new layer to your ability established. So next time you squash a bug, take a minute to reflect—you’ll arrive absent a smarter, more capable developer as a consequence of it.
Summary
Bettering your debugging techniques takes time, follow, and tolerance — however the payoff is big. It would make you a more effective, self-confident, and able developer. The next time you are knee-deep in a very mysterious bug, remember: debugging isn’t a chore — it’s an opportunity to become superior at Anything you do.